Accessing robot servo data through a C# program is a relatively straightforward process. However, in cases where a C# program with ACE integration is not available for user use, it may be necessary to resort to V+ programming.
Use DEVICES keyword to send a command to a Robot to return servo data.
Syntax
DEVICES (type, unit, error, p1, p2, ...) $out, $in
Function
Send a command or data to an external device and optionally return data. The actual operation performed depends on the device referenced.
Usage Considerations
The syntax contains optional parameters that may be useful only for specific device types and commands.
Parameters
type |
Real value, variable, or expression (interpreted as an integer) that indicates the type of device being referenced. The following types are currently available: |
unit |
Real value that indicates the device unit number. The value must be in the range 0 to (max -1), where max is the maximum number of devices of the specified type. The value should be 0 if there is only one device of the given type. |
error |
Optional real variable that receives a standard system error number if this instruction failed. If this parameter is omitted, any device error stops program execution. If the error parameter is specified, the program must check it to detect errors. The value is negative if there was an error. Otherwise, the positive value indicates the number of data bytes that were returned in the $in parameter. |
p1, p2, ... |
Optional real values that are sent to the device as part of a command. The number of values specified and the meanings of the values depend upon the particular device type. |
$out |
Optional string expression, variable, or array that defines a string value to be sent to the device as part of the command. The actual data that should be sent depends upon the device type and the command. When the $out parameter is specified as an array, the total length of the string value must be less than or equal to 520 bytes. If an array is specified, and no index is specified, element 0 is accessed first. |
$in |
Optional string variable or array that receives any data values returned from a device as the result of the command. The actual data returned depends upon the device type and the command. The error variable receives the number of input bytes returned. When the $in parameter is specified as an array, up to 512 bytes may be returned, packed in up to four successive string array elements. If an array is specified, and no index is specified, element 0 is accessed first. |
Details
DEVICES is a general-purpose instruction for accessing external devices. It is similar to the DEVICE program instruction except that data items are sent and received as strings rather than real values.
Using the information above, supply the parameters as below:
type = 3 (Robot)
unit = 1 (Robot number)
error = stt (Variable to hold the status of the command)
p1 : Select the corresponding servo data per opcode value from the table below:
Member name | Value |
Description |
MaxTorque | 1010 |
Maximum DAC value for specified joint. |
DutyCycleLimit | 1012 |
The duty cycle exceeded DAC limit. |
HarmonicDriveTorqueLimitCube | 1876 |
Harmonic Drive limit for the average cube torque. |
UnlatchedErrors | 2000 |
Unlatched error bit mask. See MotorUnlatchedErrorBits enumeration. |
LatchedErrors | 2001 |
Latched error bit mask. See MotorLatchedErrorBits enumeration. |
ServoStatus | 2002 |
Servo status bit mask. See MotorStatusBits enumeration. |
OutputLevel | 2003 |
Output level, typically a torque command between -32768 and 32767. A value of 32767 is commanding a DAC output equal to the Maximum DAC value (1010) for the specified joint. |
CommandedVelocity | 2004 |
Commanded velocity, in counts/ms. |
VelocityError | 2005 |
Velocity error (commanded minus encoder), in counts/ms. |
EncoderVelocity | 2006 |
Actual velocity, in counts/ms. |
CommandedPosition | 2007 |
Commanded position, in counts. |
PositionError | 2008 |
Position error (commanded minus encoder), in counts. |
EncoderPosition | 2009 |
Actual position, in counts. |
IndexDelta | 2012 |
Index delta (last index position minus previous index position), in counts. |
LastMotionSettlingTime | 2017 |
The motor settling time, in milliseconds, for the last motion completed. |
PeakPositionError | 2018 |
Peak Position Error. |
PeakDutyCycle | 2019 |
The peak duty cycle value. Used in conjunction with DutyCycleLevel (2243) |
CommandedAcceleration | 2027 |
Commanded acceleration, in counts/ms^2. |
VPlusCommand | 2031 |
V+ command code. |
Remarks | ||
Valid commands are: | ||
0: Current mode. Arg: DAC output. | ||
1: Free mode. Arg: DAC output. | ||
2: Position mode. Arg: Scaled position command. | ||
3: Set position. Arg: Scaled position. | ||
4: Amp command. Arg: 1 to enable, 0 to disable. | ||
5: Cal mode. Arg: None. | ||
7: Adjust position. Arg: Scaled position offset. | ||
8: Clear latched errors. Arg: Bit mask to clear. | ||
9: Drive motor. Arg: Scaled position. | ||
0xF: NOP. Arg: None. | ||
0x10: High power. Arg: 1 to turn on, 0 to turn off. | ||
0x11: Brake rel. Arg: 1 to release, 0 to engage. | ||
0x12: Velocity mode Arg: Target velocity. | ||
VPlusCommandArgument | 2032 |
V+ command argument. |
PeakTorque | 2036 |
Peak torque. |
VPlusTrajSetPoint | 2220 |
V+ Trajectory Set Point. Output from trajectory generator sent to microinterpolator each trajectory period. |
AmpBusVoltage | 2224 |
High-voltage DC bus. |
AmpTemperature | 2234 |
Amplifier temperature (degrees C). |
AmpACInputRmsVoltage | 2235 |
RMS input voltage to amplifier (volts). Note: i4L Reports 48vdc input voltage (volts). |
DutyCycleLevel | 2243 |
Filtered duty-cycle metric, used to trigger duty-cycle errors if this value exceeds the threshold. |
EncoderAlarm | 2262 |
Encoder alarm |
EncoderCommunicationError | 2263 |
Encoder communication error |
EncoderTemperature | 2265 |
Encoder temperature (degrees C). |
EStopStatus | 2268 |
E-stop status register. |
DCInputVoltage | 2285 |
DC control voltage (volts). |
BaseBoardTemperature | 2286 |
Amplifier base-board temperature (per-amp, not per-motor, degrees C). |
CurrentLoopOutput | 2287 |
The output of the 'PI' current loop. |
CurrentLoopPeakToPeakOutput | 2288 |
The peak output of the 'PI' current loop. |
BusEnergyFilter | 2289 |
Bus Energy Filter. |
PeakVelocity | 2292 |
Peak Velocity (cts/ms). |
DutyCycleLevel2 | 2303 |
Filtered duty-cycle metric, used to trigger duty-cycle errors if this value exceeds the threshold. This is used for the eV+ 2.3 or later that has three duty-cycle limit |
ForceSensorForces | 2400 |
The force sensor forces X(1), Y(2), Z(3). |
ForceSensorMoments | 2401 |
The force sensor moments Mx(1), My(2), Mz(3). |
HarmonicDriveAverageTorque | 2809 |
Harmonic Drive average torque. |
HarmonicDriveLife | 2810 |
Harmonic Drive life usage. |
Sample V+ Program to read the Duty Cycle and Temperature of the Robot's motors:
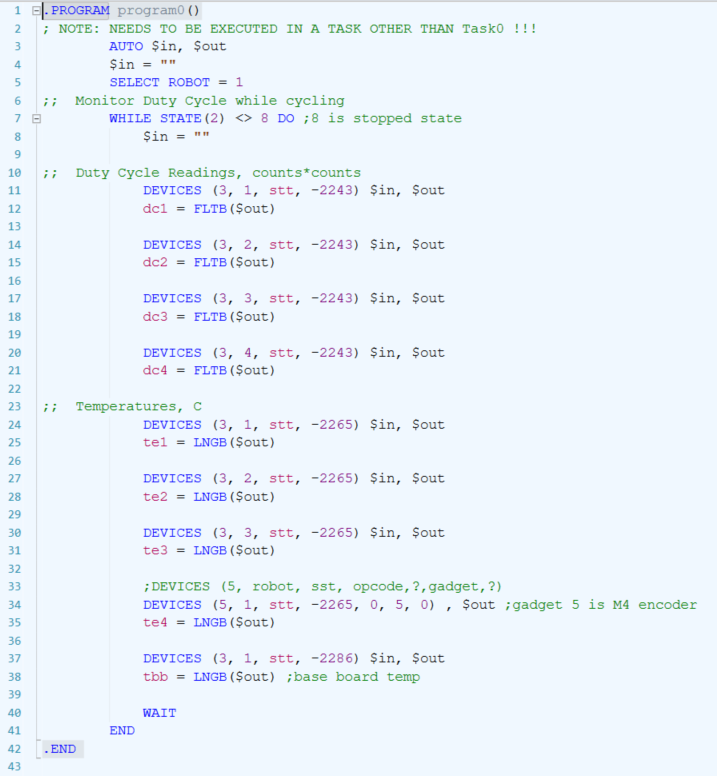